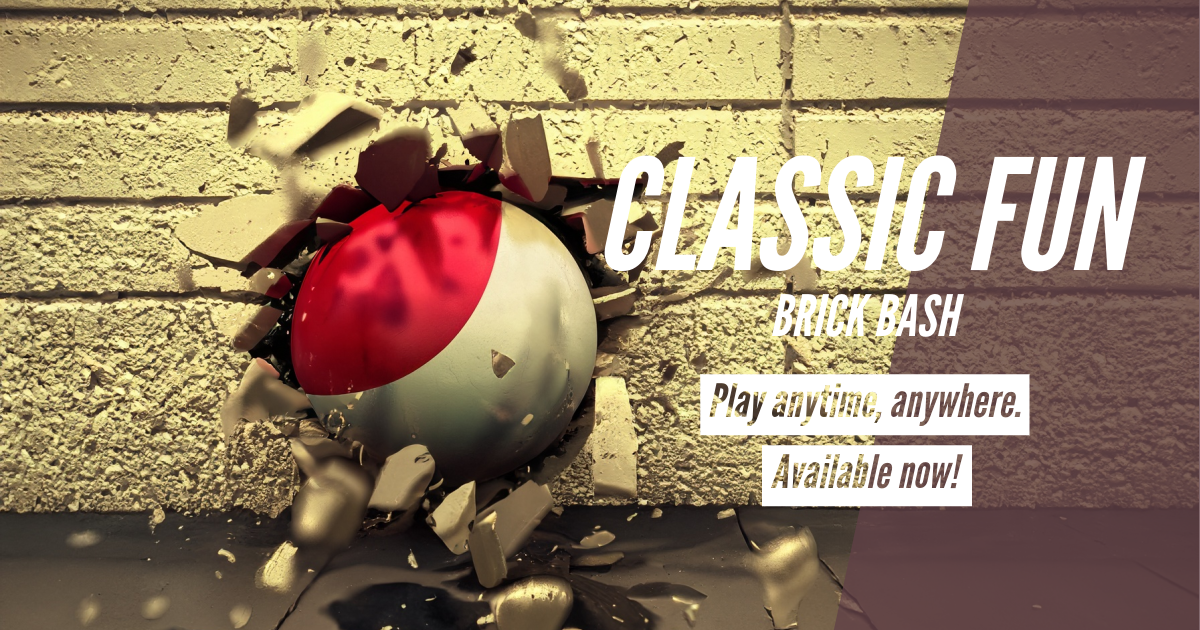
Brick Breaker games require bricks, a paddle, and a ball to set up a play area. A paddle controls the ball’s bounce upwards, aiming to break bricks and clear levels. A basic HTML, CSS, and JavaScript framework will be provided. By using responsive design and mobile development frameworks, it will focus on web development aspects for later adaptation to mobile devices.
Step 1: HTML Structure
The game area, bricks, paddle, and ball should be defined.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Brick Breaker Game</title>
<link rel="stylesheet" href="style.css">
</head>
<body>
<div id="gameArea">
<div id="paddle"></div>
<div id="ball"></div>
</div>
<script src="game.js"></script>
</body>
</html>
Step 2: CSS Styling
Make sure the game area, paddle, ball, and bricks all have basic styles. For simplicity, bricks will be added dynamically with JavaScript.
#gameArea {
width: 400px;
height: 600px;
position: relative;
margin: 20px auto;
background-color: #000;
overflow: hidden;
}
#paddle {
width: 80px;
height: 20px;
position: absolute;
bottom: 20px;
left: 50%;
transform: translateX(-50%);
background-color: #fff;
}
#ball {
width: 20px;
height: 20px;
position: absolute;
bottom: 50px;
left: 50%;
transform: translateX(-50%);
background-color: #fff;
border-radius: 50%;
}
Step 3: JavaScript Logic
Move the paddle, bounce the ball, and break bricks to implement the logic of the game.
document.addEventListener('DOMContentLoaded', () => {
const gameArea = document.getElementById('gameArea');
const paddle = document.getElementById('paddle');
const ball = document.getElementById('ball');
let ballDirectionX = 2;
let ballDirectionY = 2;
// Move paddle
document.addEventListener('mousemove', (e) => {
let gameAreaBounds = gameArea.getBoundingClientRect();
let paddlePosition = e.clientX - gameAreaBounds.left;
if (paddlePosition < gameAreaBounds.width - paddle.offsetWidth && paddlePosition > 0) {
paddle.style.left = paddlePosition + 'px';
}
});
// Ball movement
function moveBall() {
let ballCurrentX = ball.offsetLeft + ballDirectionX;
let ballCurrentY = ball.offsetTop + ballDirectionY;
// Ball boundary checks
if (ballCurrentX >= gameArea.offsetWidth - ball.offsetWidth || ballCurrentX <= 0) {
ballDirectionX *= -1;
}
if (ballCurrentY >= gameArea.offsetHeight - ball.offsetHeight || ballCurrentY <= 0) {
ballDirectionY *= -1;
}
ball.style.left = ballCurrentX + 'px';
ball.style.top = ballCurrentY + 'px';
requestAnimationFrame(moveBall);
}
moveBall();
});
Game mechanics explained
Game Area: This is the space where the game takes place. The rectangular box contains a paddle, a ball, and bricks.
Paddle: The player moves the paddle left and right (in this case, using the mouse). The goal is to keep the ball in play and direct it towards the bricks.
Ball: The ball moves automatically, bouncing off the game area walls and the paddle. When the ball hits a brick, the brick should disappear (logic not included in this simple version).
Bricks: Bricks are arranged at the top of the game area. The objective is to destroy all bricks by hitting them with the ball to clear the level.
Enhancements for a Complete Game
To make this a fully functional Brick Breaker game, consider adding:
Brick Logic: Generate bricks dynamically and remove them when hit by the ball.
Scoring: Implement a scoring system, awarding points for each brick broken.
Levels: Create levels with different brick arrangements and increasing difficulty.
Lives: Give the player a certain number of lives, decreasing one each time the ball falls below the paddle.
Summary
This setup gives you a basic framework for a Brick Breaker game. While it covers fundamental aspects like moving the paddle and bouncing the ball, a complete game would include additional features such as breaking bricks, scoring, and advancing through levels. This project can be a fun introduction to game development with web technologies, with plenty of opportunities for creativity and expansion.
Setting Up the Game Environment
HTML Structure: This is like drawing the playground where our game will happen. We have a “game,” which is the field, a “paddle,” which is like our bat, and a “ball,” which we will use to hit bricks.
CSS Styling: This step is like painting our playground, bat, and ball. We make the game area into a rectangle, color it black, and place it in the middle of the screen. The paddle and ball have a white color for contrast, with the paddle at the bottom and just above it.
Making the game work
JavaScript Logic: Here’s where we add rules and actions to our game, making it interactive and fun.
Moving the paddle
We let the player move the paddle left and right using the mouse. When you shift your mouse across the screen, the paddle follows your movement but stays within the game area.
Bouncing the ball
The ball starts moving by itself. It bounces off the game area walls and the paddle. If it hits the sides of the game area, it rebounds back in the opposite direction. If it hits the top or the paddle, it bounces back.
The ball keeps moving; you must hit it with the paddle whenever it comes down.
How the Game Plays Out
Objective: Your goal is to use the ball to break all the bricks arranged at the top of the game area. In our simple setup, we still need to add bricks, but imagine there are invisible targets you’re aiming for.
Win the Game: You win by breaking all the bricks with the ball. In a more advanced version of the game, this would increase your score and take you to the next level. This would be done with more bricks or a faster ball.
Critical Parts of the Code Explained
Game Area: The big black rectangle where our game takes place.
Paddle Movement: We track where the mouse is and move the paddle to that position, ensuring it stays within the game area.
Ball Movement: The ball moves straight until it hits a boundary or the paddle, then bounces off. We calculate its updated direction each time it bounces.
Enhancements for a Full Game
To make this a complete Brick Breaker game, we need:
Add bricks at the top of the game area for the ball to break.
Create a way for the ball to “destroy” a brick when it hits, maybe making it disappear or change color.
Keep score of how many bricks you’ve broken and you may lose a life if the ball gets past your paddle.
Summary for students
Creating a Brick Breaker game involves setting up a play area, controlling a paddle to bounce a ball, and breaking bricks. It combines elements of art (drawing and coloring the game) and rules (making the paddle and ball move as expected) to create a fun and interactive experience.
Reference Links to Include:
Mobile Game Development Tools:
- An introduction to tools and frameworks specifically suited for developing mobile games, including Unity and Unreal Engine.
- Suggested Search: “Best tools for mobile game development”
- For comprehensive guides on using Unity for game development, suitable for brick breaker games on mobile platforms.
Mobile Game Development Tutorials:
- To offer step-by-step instructions and best practices for creating engaging mobile games, including genre-specific tips.
- Suggested Search: “Mobile game development tutorials for beginners”
Stack Overflow for Mobile Game Development:
- A resource for troubleshooting development issues and learning from the experiences of other game developers.
Leave a Reply