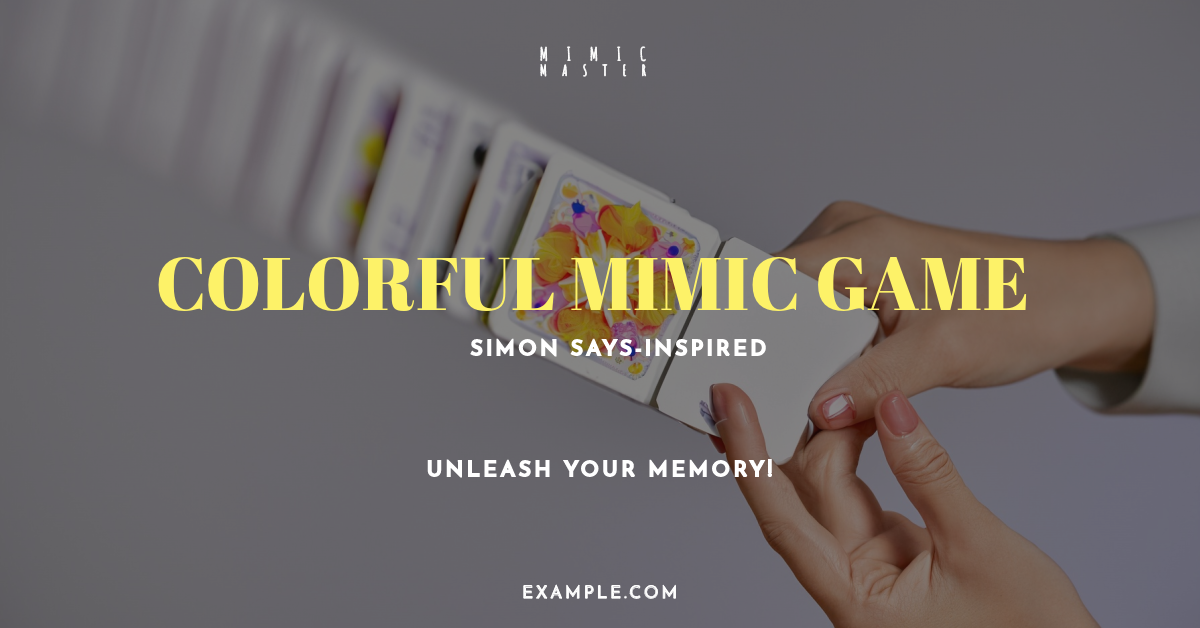
The goal of creating a “Simon Says” game is to generate a sequence of colors or patterns that players must memorize and repeat. As the game progresses, this sequence becomes more complex, testing the player’s memory skills. This explanation will guide you through setting up a basic game version using HTML, CSS, and JavaScript.
Step 1: HTML Structure
Four colored panels representing sequence elements and a start button should define the game area.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Simon Says Game</title>
<link rel="stylesheet" href="style.css">
</head>
<body>
<div id="gameArea">
<div class="colorPanel" id="greenPanel"></div>
<div class="colorPanel" id="redPanel"></div>
<div class="colorPanel" id="yellowPanel"></div>
<div class="colorPanel" id="bluePanel"></div>
</div>
<button id="startButton">Start Game</button>
<script src="game.js"></script>
</body>
</html>
Step 2: CSS Styling
Style the game area and color the panels. The panels should visually indicate when they are part of a sequence.
#gameArea {
display: flex;
flex-wrap: wrap;
width: 200px;
height: 200px;
margin: auto;
}
.colorPanel {
width: 50%;
height: 50%;
opacity: 0.5; /* Panels appear dimmed by default */
}
#greenPanel { background-color: green; }
#redPanel { background-color: red; }
#yellowPanel { background-color: yellow; }
#bluePanel { background-color: blue; }
.colorPanel.active {
opacity: 1; /* Panels appear fully colored when active */
}
Step 3: JavaScript Logic
Implement the game logic: generate a sequence, allow the player to input a sequence, and check for matches.
const panels = document.querySelectorAll('.colorPanel');
const startButton = document.getElementById('startButton');
let sequence = [];
let playerSequence = [];
let sequenceIndex = 0;
// Start the game
startButton.addEventListener('click', startGame);
function startGame() {
sequence = []; // Reset sequence for a new game
addSequenceColor();
}
function addSequenceColor() {
const colors = ['green', 'red', 'yellow', 'blue'];
const randomColor = colors[Math.floor(Math.random() * colors.length)];
sequence.push(randomColor);
playSequence();
}
function playSequence() {
sequenceIndex = 0;
sequence.forEach((color, index) => {
setTimeout(() => {
activatePanel(color);
}, 1000 * (index + 1));
});
}
function activatePanel(color) {
const panel = document.getElementById(color + 'Panel');
panel.classList.add('active');
setTimeout(() => {
panel.classList.remove('active');
}, 500);
}
panels.forEach(panel => {
panel.addEventListener('click', () => {
const color = panel.id.replace('Panel', '');
playerSequence.push(color);
checkSequence(playerSequence.length - 1);
});
});
function checkSequence(index) {
if (playerSequence[index] === sequence[index]) {
if (playerSequence.length === sequence.length) {
playerSequence = []; // Reset player's sequence
addSequenceColor(); // Add a new color to the sequence
}
} else {
alert('Game over! Wrong sequence.');
// Reset for a new game or end the game
}
}
Game mechanics explained
Game Start: The game begins with an empty sequence when the player clicks the “Start Game” button.
Sequence Generation: Random colors are added to the sequence, which the game displays individually by making the corresponding panels light up.
Player Input: The player clicks on the panels to replicate the sequence, and each click adds to the player’s input sequence.
Checking Matches: After each player inputs, the game checks if the player’s sequence matches the generated sequence up to that point. If the player completes the sequence correctly, another color is added, making the sequence longer. If the player makes a mistake, the game ends.
Summary
This “Simon Says” game challenges players to memorize and replicate an increasingly complex sequence of colors. The game setup involves:
I am creating a visual interface with colored panels.
I generate random sequences of these colors.
They allow the player to interact with the panels to repeat the sequence.
JavaScript handles the game’s logic, tracking sequence and player inputs and determining game outcomes based on player accuracy.
The game setup
HTML Structure: This is like a game board. We have four colored squares (panels) and a start button. Each square is a part of the game where you must pay attention to the sequence.
CSS Styling: This step makes our game look nice. The squares are styled with different colors (green, red, yellow, blue), and they’re faded out (opacity: 0.5) until they become part of the sequence – then they light up (opacity: 1).
JavaScript Logic: This is where game rules and actions are defined.
Start the game.
When you click the “Start Game” button, a new game starts. The game begins with no sequence, and then we add the first color to the sequence.
Added Colors to the Sequence
The game randomly picks a color and adds it to the sequence. This sequence is what you need to remember and repeat.
Showing the sequence.
The game shows you the sequence by lighting up the colored panels individually. Each color in the sequence makes its corresponding panel light up briefly.
Repeat the sequence.
Now it’s your turn. You repeat the sequence by clicking on the colored panels in the order shown.
Each time you click a panel, the game checks if you have followed the sequence correctly.
Check Your Input
As you click on the panels, the game compares your clicks with the sequence it generated.
If you’re correct and complete the whole sequence, the game adds another color, making it longer and more challenging.
If you make a mistake, it’s game over. The game tells you you’ve clicked on the wrong sequence.
What Makes the Game Work?
Sequence Array: This keeps track of the game’s color sequence. It starts empty and grows longer as repeated.
PlayerSequence Array: This records the colors you click to repeat the sequence.
Random Color Selection: The game randomly picks out a color and shows it to you by lighting up the corresponding panel.
User Interaction: You interact with the game by clicking on the colored panels and matching the sequence shown in the game.
Feedback: The game immediately lets you know if you’re following the sequence correctly or made a mistake.
Summary for students
Imagine playing a memory game where you watch a light show and then try to repeat it exactly as you saw it. The show becomes longer and more challenging each time you get it right. But if you call it wrong, you have to start over. This game tests how well you remember and repeat patterns.
Reference Links to Include:
Game Development with Python:
- For tutorials and guides on using Python for creating games, including similar to Simon Says.
- Suggested Search: “Python game development tutorials”
Pygame Documentation:
- If your Simon Says-style game uses Pygame, this documentation is invaluable for adding graphics and interactivity.
Interactive Game Design Principles:
- To offer insights into crafting engaging and intuitive game interfaces and experiences.
- Suggested Search: “Principles of interactive game design”
GitHub Repositories for Simon Says Games:
- For examples of Simon Says games coded by others, which can offer inspiration and coding insights.
Leave a Reply