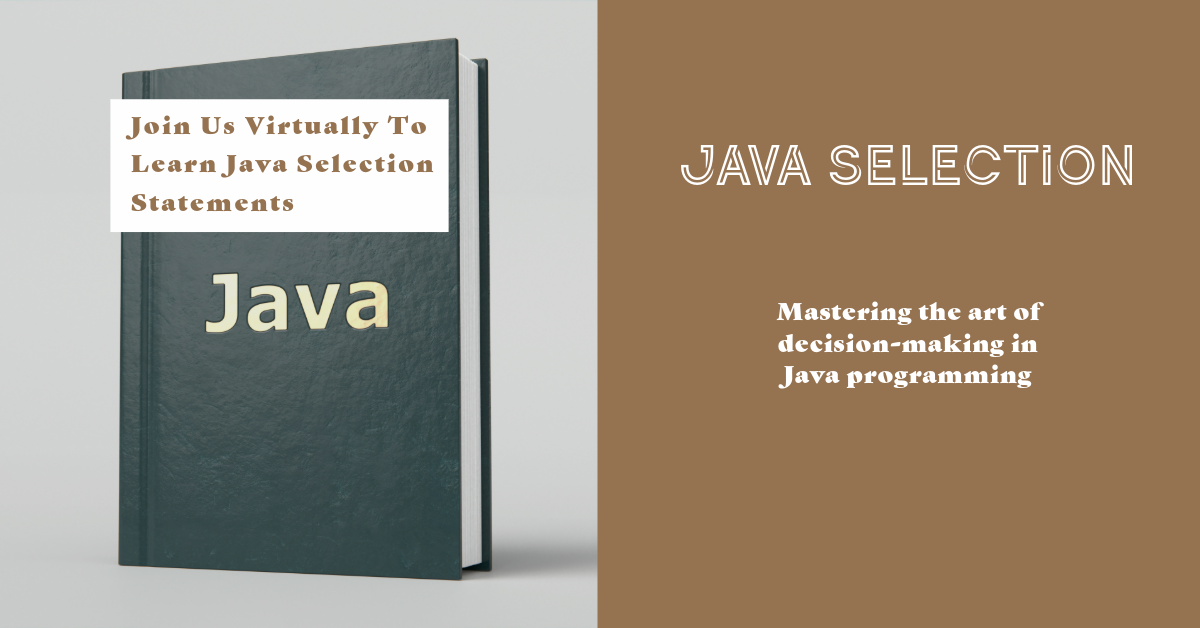
Java selection statements control the execution flow based on conditions. Java selection statements are:
If-else statement
Switch statement
If-else Statement
The if-else statement lets you run particular code blocks based on a Boolean condition. If the condition evaluates to true, the block inside the if part executes. Otherwise, the code inside the else part (if present) executes.
Example Problem: Checking if a number is positive or negative.
public class PositiveNegative {
public static void main(String[] args) {
int number = -5;
if (number > 0) {
System.out.println(number + " is positive.");
} else if (number < 0) {
System.out.println(number + " is negative.");
} else {
System.out.println("The number is zero.");
}
}
}
Using the if-else statement, this program checks if a number is positive or negative.
Switch statement
The switch statement allows you to select one of many code blocks to be executed based on the value of a variable or expression. It’s a cleaner alternative to using multiple if-else statements when comparing the same variable against multiple values.
Example Problem: Day of the Week
public class DayOfWeek {
public static void main(String[] args) {
int day = 4; // 1: Monday, 2: Tuesday, ..., 7: Sunday
switch (day) {
case 1:
System.out.println("Monday");
break;
case 2:
System.out.println("Tuesday");
break;
case 3:
System.out.println("Wednesday");
break;
case 4:
System.out.println("Thursday");
break;
case 5:
System.out.println("Friday");
break;
case 6:
System.out.println("Saturday");
break;
case 7:
System.out.println("Sunday");
break;
default:
System.out.println("Invalid day");
}
}
}
This program uses the switch statement to print the name of the day of the week based on its numerical value.
Conclusion
Java if-else and switch statements control execution flow based on conditions or values. The choice between using an if-else statement or a switch statement often depends on your program’s specific needs and code clarity.
Reference Links to Include:
Oracle’s Java Documentation:
- For official and comprehensive documentation on Java selection statements.
Java Programming Tutorials by Oracle:
- Offers in-depth tutorials on Java programming constructs, including selection statements.
GitHub Java Projects:
- For practical examples of Java code utilizing selection statements in real-world applications.
Stack Overflow for Java Programming Questions:
- A valuable resource for troubleshooting and learning from the Java programming community’s questions and answers on selection statements.
Leave a Reply