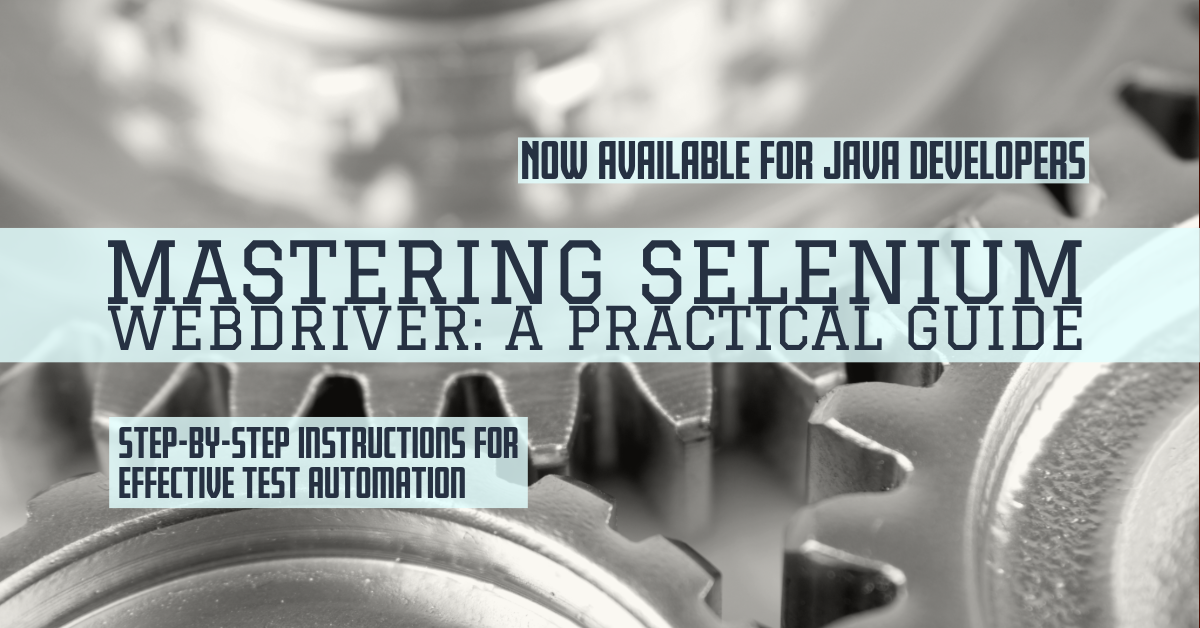
Selenium WebDriver in a Java environment utilizes JavaScript. This is especially useful when interacting with elements difficult to access through the standard WebDriver API. It can be done via the JavascriptExecutor interface. This allows you to execute JavaScript code in the context of the currently selected frame or window.
Here’s a step-by-step guide on how to use JavaScript within Selenium WebDriver in Java:
1. Setup WebDriver
First, ensure you have Selenium WebDriver set up in your Java project. You’ll need the Selenium Java client and drivers for the browsers you intend to automate. If you’re using Maven, include the Selenium dependency in your pom.xml.
<dependency>
<groupId>org.seleniumhq.selenium</groupId>
<artifactId>selenium-java</artifactId>
<version>3.141.59</version> <!-- Use the latest version -->
</dependency>
2. Initialize WebDriver and Navigate to a Web Page
Initialize your WebDriver and navigate to the web page where you want to execute JavaScript.
WebDriver driver = new ChromeDriver(); // Ensure you have the ChromeDriver executable in your PATH
driver.get("https://www.example.com");
3. Execute JavaScript
To execute JavaScript, you must cast your driver to JavascriptExecutor and call the executeScript method.
JavascriptExecutor js = (JavascriptExecutor) driver;
js.executeScript("alert('Hello, World!');");
Example Use Cases
Scroll to an element
JavaScript can help scroll to elements not visible in the viewport.
JavascriptExecutor js = (JavascriptExecutor) driver;
WebElement element = driver.findElement(By.id("someId"));
js.executeScript("arguments[0].scrollIntoView(true);", element);
Getting the Value of an Element’s Attribute
When the WebDriver API doesn’t provide a direct way to get the value of an element’s attribute, you can use JavaScript.
JavascriptExecutor js = (JavascriptExecutor) driver;
String value = (String) js.executeScript("return document.getElementById('someId').getAttribute('attributeName');");
Set the value of an input field
Directly setting the value of an input field is more reliable than simulating keyboard input.
JavascriptExecutor js = (JavascriptExecutor) driver;
js.executeScript("document.getElementById('textInput').value = 'New Value';");
Handling Return Value
The executeScript method returns an Object. You can cast this return value to the expected type. For instance, you can cast the result to Long if your JavaScript expression returns a number.
long height = (Long) js.executeScript("return window.innerHeight;");
Considerations
Asynchronous JavaScript: If you’re running asynchronous JavaScript, use the executeAsyncScript method instead of executeScript. You’ll need to manage the script’s callback to signal completion.
Cross-Browser Compatibility: Ensure your JavaScript code is compatible with all browsers you plan to automate, as JavaScript execution can differ across browsers.
Using JavaScript with Selenium WebDriver extends your automation capabilities, allowing you to perform actions that the WebDriver API might not directly support. However, it should be used judiciously, as it can make your tests more dependent on the internal structure of the web page, potentially leading to brittle tests.
For reference links and additional learning, although I can’t provide live internet links, consider exploring:
- Selenium Official Documentation : Offers comprehensive guides on using Selenium WebDriver, including integration with JavaScript in Java projects.
Leave a Reply