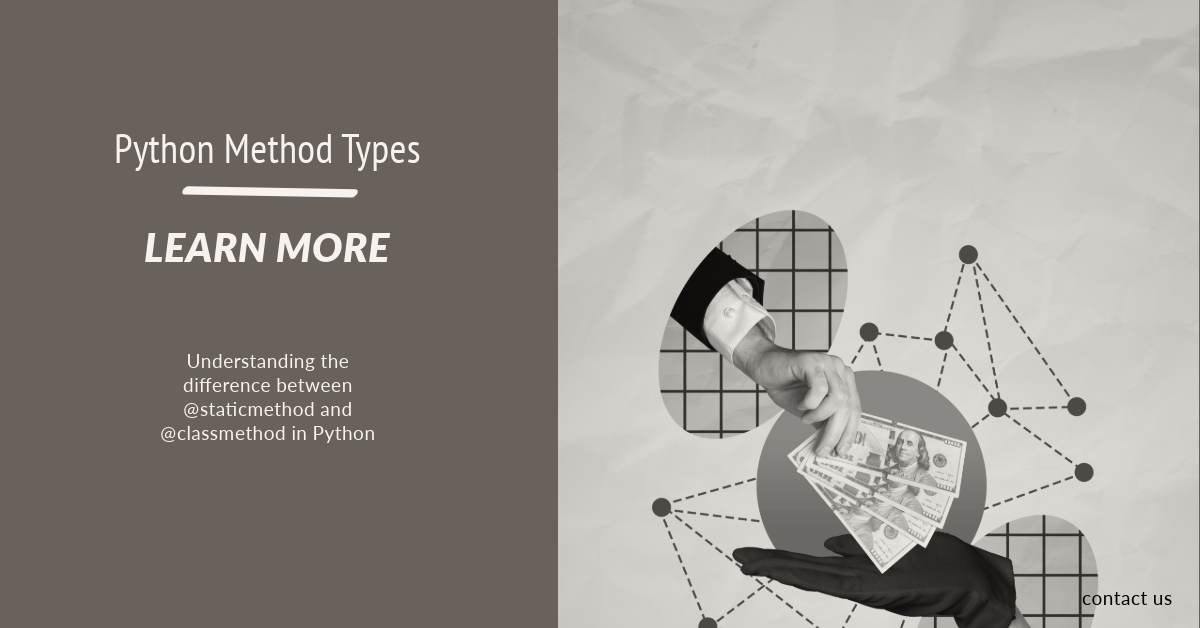
In Python, @staticmethod and @classmethod are decorators that allow you to define methods within a class scope that are not bound to a class instance. The two serve different purposes and behave differently, despite their similarity.
@staticmethod
A static method knows nothing about the class or instance it was called from. It just gets the arguments passed, with no implicit first argument like self (the instance) or cls (the class). It behaves like a plain function that lives in a class body. It’s defined using the @staticmethod decorator.
Example of the @staticmethod:
class MyClass:
@staticmethod
def my_static_method(arg1, arg2):
return arg1 + arg2
# Static methods can be called on a class...
print(MyClass.my_static_method(5, 3)) # Output: 8
# ... or on an instance
instance = MyClass()
print(instance.my_static_method(10, 20)) # Output: 30
@classmethod
On the other hand, a class method gets passed the class it was called on or the class of the instance it was called on. This is the first argument. By convention, it is usually named CLS. This means you can use the class and its properties within your method. It’s defined using the @classmethod decorator.
Example of the @classmethod:
class MyClass:
class_var = 10
@classmethod
def my_class_method(cls, arg1):
return cls.class_var + arg1
# Class methods can be called on a class...
print(MyClass.my_class_method(5)) # Output: 15
# ... or on an instance
instance = MyClass()
print(instance.my_class_method(20)) # Output: 30
Key differences
Binding: @staticmethod does not bind to the instance or class, meaning it doesn’t take a self or CLS parameter, whereas @classmethod binds to the class, taking a CLS parameter.
Usage: Use @staticmethod when you need a utility function that doesn’t access class or instance properties. Use @classmethod when you need to access or modify the class state or call other class methods from within this method.
Inheritance: With @classmethod, subclasses that override class variables or methods can affect the behavior of the class method because the process receives the class it was called on. It can benefit factory methods that instantiate class instances. With @staticmethod, inheritance doesn’t impact the method’s behavior since it doesn’t know the class hierarchy.
Choosing between @staticmethod and @classmethod depends on whether you need to access or modify the class state. It also depends on the specific design of your classes and their intended use cases.
Reference Links:
- Real Python Article: Python Decorators
Leave a Reply