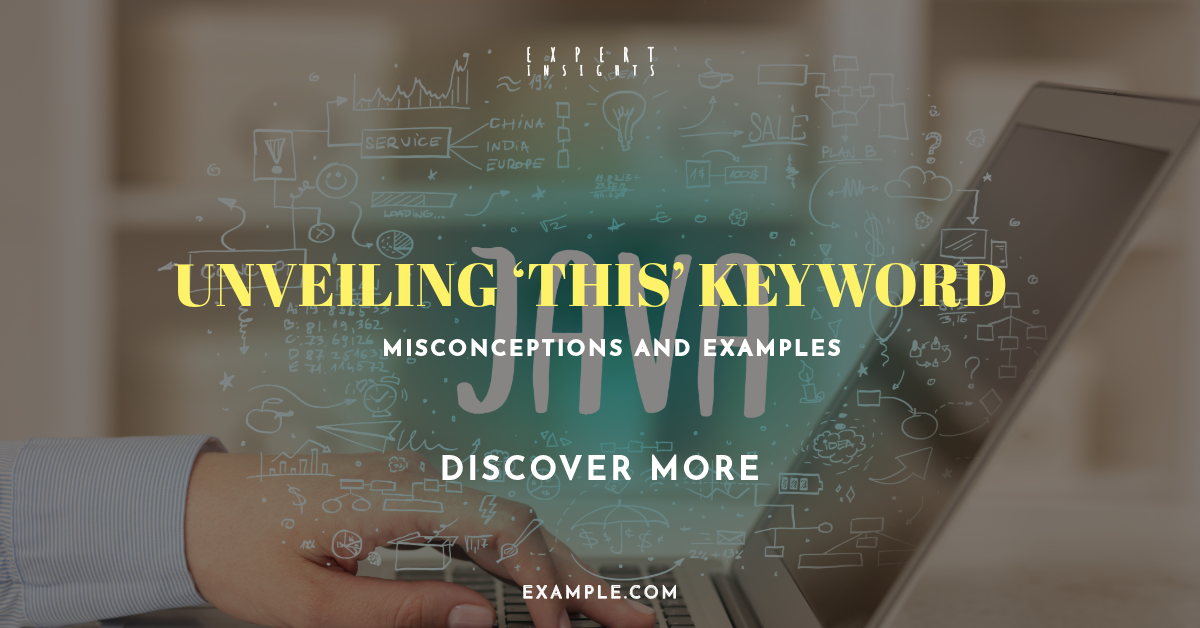
In Java, this keyword is used for several specific purposes related to the current instance of a class. Here are the common uses of this:
To refer to the current instance’s variables (especially to distinguish instance variables from method parameters).
To invoke the current instance’s methods.
To pass the current instance as an argument in method calls.
To invoke the current class’s constructor.
Given these uses, let’s clarify what this is not used for in Java with an example for better understanding.
What this is Not Used For
This is not used to refer to constant variables or methods. This context belongs to the class rather than any instance, so using this to access static members is incorrect and unnecessary. Instead, you should utilize or omit the class name when accessing static members from within the same class.
Example Code Demonstrating Incorrect Use:
public class Example {
static int staticVar = 10;
int instanceVar = 20;
public void myMethod() {
// Correct: Referring to instance variable
System.out.println(this.instanceVar); // Outputs: 20
// Incorrect: Using 'this' to refer to a static variable
// Correct way is simply using the variable name or class name
// System.out.println(this.staticVar); // This is not correct
System.out.println(staticVar); // Correct for static variables
}
public static void staticMethod() {
// Incorrect: 'this' cannot be used in static context
// System.out.println(this.instanceVar); // Compilation error
// Correct: Accessing static variable
System.out.println(staticVar); // Outputs: 10
}
public static void main(String[] args) {
new Example().myMethod();
staticMethod();
}
}
In the method ‘instance’, using this.instanceVar is correct and refers to the instance variable instanceVar. However, referring to the constant variable staticVar with this.staticVar is not the correct use, as staticVar belongs to the class level, not any particular instance. The proper way to access static variables is by their name or example. Static var if accessed from outside the class where it’s defined.
In the static method, using this results in a compilation error. This is because this refers to the current object, and there is no current object in a static context.
Conclusion
This keyword is not used for accessing class static members (variables or methods). Static members belong to the class as a whole, not to any specific instance. They are accessed using the class name or directly without a prefix if called from within the same class.
Reference Links to Include:
Oracle’s Java Documentation:
- For official and comprehensive explanations on the ‘this’ keyword and its scope within Java programming.
Java Tutorials and Best Practices:
- Offers insights into effective Java programming, including the use of the ‘this’ keyword.
- Suggested Search: “Effective Java programming practices”
GitHub Java Projects:
- To provide real-world examples of the ‘this’ keyword in action within Java applications.
Stack Overflow for Java Programming:
- A resource for troubleshooting and learning from community discussions related to the ‘this’ keyword and other Java programming topics.
Leave a Reply